1.1. 字符流
字符流只能操作文本文件,不可操作图片。
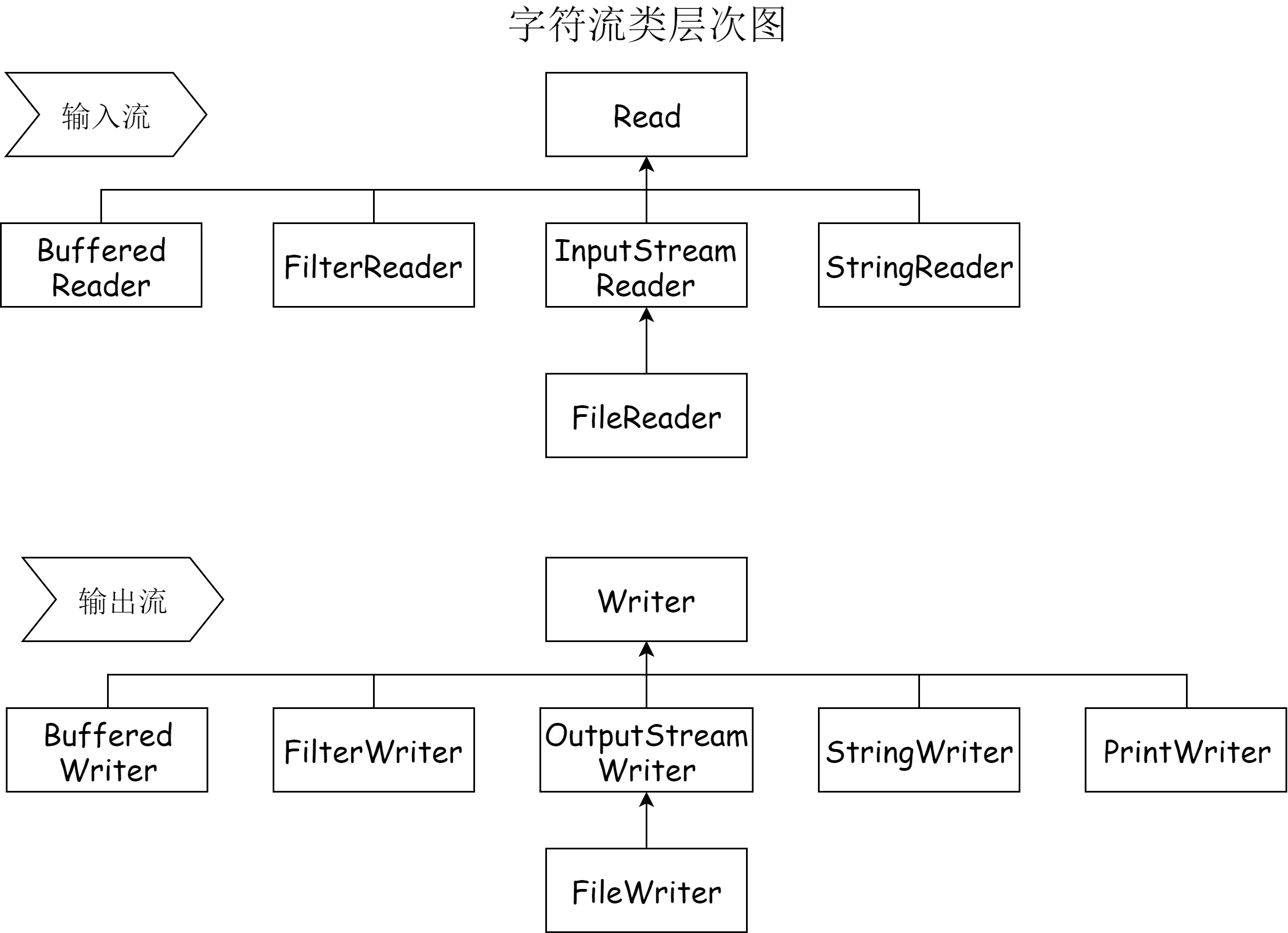
2. 字符流的父类(抽象类)
Reader:字符输入流
public int read()
从流中读取单个字符,用整型来返回读取的字符;当读到流底部时返回-1。
public int read(char[] c)
从流中读取字符保存到c数组中,返回读取的字符个数,当读到流底部时返回-1。
public int read(char[] cbuf,int off,int len){}
抽象方法。
Writer:字符输出流
public void write(int n)
写入单个字符,只能写入包含16位低阶字节的整型数值,16位高阶字节将会被忽略。
public void write(String str)
写入一个字符串。
public void write(char[] cbuf)
写入一个字符数组。
3. 字符流的子类
FileReader:
public int read()
继承自InputStreamReader类。读取单个字符,返回读取的字符,当读到流底部时返回-1。
public int read(char[] c)
继承自Reader类。
public int read(char[] cbuf,int offset,int length)
继承自InputStreamReader类。从流中读取部分字符到cbuf中指定位置,返回读取到的字符个数,当读到流底部时返回-1。
String path = "/Users/admin/Downloads/data.txt";
FileReader fr = new FileReader(path);
// 读取单个字节
// int data = 0;
// while ((data = fr.read()) != -1) {
// System.out.print((char) data);
// }s
// 读取多个字节
char[] data = new char[1024];
int count = 0;
while ((count = fr.read(data)) != -1) {
System.out.println(new String(data, 0, count));
}
fr.close();
FileWriter:
public void write(int c)
继承自OutputStreamWriter类,写入一个字符。
public void write(String str)
继承自Writer类。
public void Write(char[] cbuf)
继承自Writer类。
String path = "/Users/admin/Downloads/data.txt";
FileWriter fw = new FileWriter(path);
for (int i = 0; i < 10; i++) {
fw.write("Java是世界上最好的语言\r\n");
fw.flush();
}
fw.close();
4. 案例
String path = "/Users/admin/Downloads/data.txt";
String path1 = "/Users/admin/Downloads/data1.txt";
FileReader fr = new FileReader(path);
FileWriter fw = new FileWriter(path1);
int data = 0;
while ((data = fr.read()) != -1) {
fw.write(data);
}
fr.close();
fw.close();
4.1. 字符缓冲流
- 缓冲流:BufferedReader/BufferedWriter
- 高效读写
- 支持换行输入符
- 可一次写一行、读一行。
- 常用方法
readLine()
读取一行的内容newLine()
写入一个换行符,换行符的内容由操作系统给定。其中,Windows系统为\r\n
,Unix/Linux系统为\n
。
使用字符缓冲流读取文件
String path = "/Users/admin/Downloads/data.txt";
FileReader fileReader = new FileReader(path);
BufferedReader bufferedReader = new BufferedReader(fileReader);
// 按字符读取
// char[] chars = new char[1024];
// int count = 0;
// while ((count = bufferedReader.read(chars)) != -1) {
// System.out.println(new String(chars, 0, count));
// }
// 按行读取
String str = null;
while ((str = bufferedReader.readLine()) != null) {
System.out.println(str);
}
bufferedReader.close();
使用字符缓冲流写入文件
String path = "/Users/admin/Downloads/data.txt";
FileWriter fw = new FileWriter(path);
BufferedWriter bw = new BufferedWriter(fw);
for (int i = 0; i < 10; i++) {
bw.write("好好学习,天天向上");
bw.newLine(); // 新增一个换行符
bw.flush();
}
bw.close();
4.2. 打印流
- PrintWriter:
- 封装了print()/println()方法,支持写入后换行。
- 支持数据原样打印。
String path = "/Users/admin/Downloads/data.txt";
PrintWriter printWriter=new PrintWriter(path);
//打印到文件
printWriter.println(97);//97
printWriter.println('b');//b
printWriter.println(3.14);//3.14
printWriter.println(true);//true
printWriter.close();
4.3. 转换流
- 桥转换流:InputStreamReader/OutputStreamWriter
- 可将字节流转换为字符流。
- 可设置字符的编码方式。
String path = "/Users/admin/Downloads/data.txt";
FileInputStream fis = new FileInputStream(path);
InputStreamReader ism = new InputStreamReader(fis, "utf-8");
char[] chars = new char[1024];
int count = 0;
while ((count = ism.read(chars))!= -1){
System.out.println(new String(chars, 0, count));
}
ism.close();
String path = "/Users/admin/Downloads/data.txt";
FileOutputStream fos = new FileOutputStream(path);
OutputStreamWriter osw = new OutputStreamWriter(fos, "gbk");
for (int i = 0; i < 10; i++) {
osw.write("hello, world!\r\n");
osw.flush();
}
osw.close();